pandasを使って、1億23万456円といった金額の表記を、100230456といった数値に変換します。数字が漢数字の場合には対応していません。
もくじ
億万円を数値に変換
- 億円を万円に変換
- ●億●万円を万円に変換
- ●億●万●円を●万●円に変換
- ●万円を●万0円に変換
- すべての金額を数値に
このように、5段階の処理で億万円表記の金額をpandasで数値に変換していきます。
処理の順番を変えると上手くいかないので、1番から順に処理してください。
4番までは、すべてstr
にしないとエラーがでます。
文字だけで説明すると分かりにくいので、jupyter notebookで処理順に結果を示しながら説明します。
pandasをインストールしていない場合は、インストールしてください。
pip install pandas
ソースコード
import pandas as pd
df = pd.DataFrame({'A': ['11億円','22億222万円','33億3333万3333円','4444万円','5555万5555円']})
df2 = df['A'].apply(lambda x: x.strip('億円') + '0000万円' if '億円' in x else x)
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万円')[0])) + '万円' if '億' in x and '万円' in x else x)
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万')[0])) + '万' + str(int(x.split('億')[1].split('万')[1].strip('円'))) + '円' if '億' in x else x)
df2 = df2.apply(lambda x: x.split('万円')[0] + '万' + '0円' if '万円' in x else x)
df['B'] = df2.apply(lambda x: int(x.split('万')[0]) * 10000 + int(x.split('万')[1].strip('円')) if '万' in x else x)
DataFrameを作成
import pandas as pd
df = pd.DataFrame({'A': ['11億円','22億222万円','33億3333万3333円','4444万円','5555万5555円']})
どのように処理されるのか検証するために、数字は全角と半角を混ぜて作成しています。
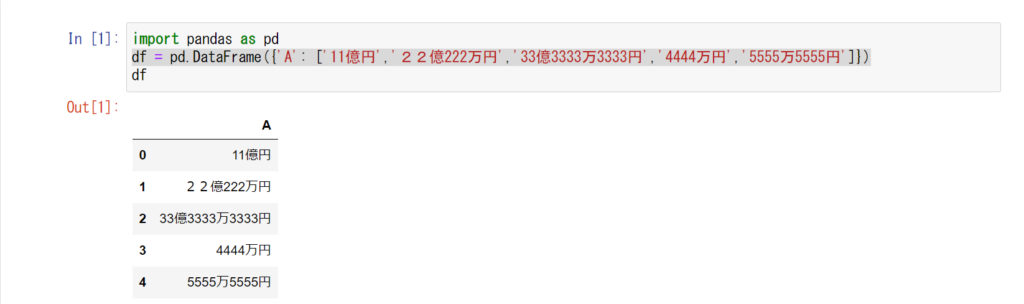
億円 ⇒ 万円
df2 = df['A'].apply(lambda x: x.strip('億円') + '0000万円' if '億円' in x else x)
億円で終了しているものに0000万円を追加して、万円表記になるようにします。
最初に作成したDataFrameに処理したものを順次代入していくと、間違っていても分かりにくくなるので、最後にカラム’B’を作成して変換した数値をすべて代入します。

●億●万円 ⇒ 万円
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万円')[0])) + '万円' if '億' in x and '万円' in x else x)
●億●万円で終了しているものを、万円表記にします。
億円で終了しているものは前回で処理しているので、これで億が入っているのは万未満の数字があるものだけになります。

●億●万●円 ⇒ ●万●円
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万')[0])) + '万' + str(int(x.split('億')[1].split('万')[1].strip('円'))) + '円' if '億' in x else x)
ここで億が入っているものをすべて万表記にします。

●万円 ⇒ ●万0円
df2 = df2.apply(lambda x: x.split('万円')[0] + '万' + '0円' if '万円' in x else x)
万円で終了しているものを、万0円に変換することで数値に変換しやすくします。

すべてを数値に変換
df['B'] = df2.apply(lambda x: int(x.split('万')[0]) * 10000 + int(x.split('万')[1].strip('円')) if '万' in x else x)
最初に作成したDataFrameに新しいカラム’B’を作成し、金額を数値に変換したものを全部代入して完成です。
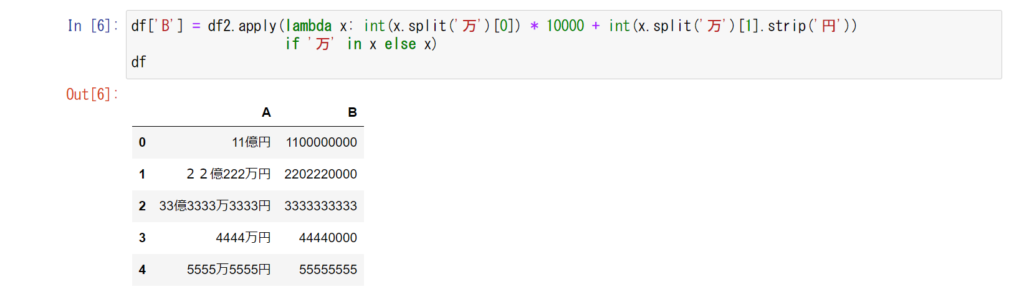
修正:ソースコード
22億222円といった万の位がないデータが、扱っているデータの中に無かったため見落としていました。万の位がないデータに対応するために、ソースコードを少し修正しました。
import pandas as pd
df = pd.DataFrame({'A': ['11億円','22億222円','33億333万円','44億4444万4444円','5555万円','6666万6666円','7777円']})
df2 = df['A'].apply(lambda x: x.strip('億円') + '0000万円' if '億円' in x else x)
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000) + '万' + x.split('億')[1] if '億' in x and '万' not in x else x)
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万円')[0])) + '万円' if '億' in x and '万円' in x else x)
df2 = df2.apply(lambda x: str(int(x.split('億')[0]) * 10000 + int(x.split('億')[1].split('万')[0])) + '万' + str(int(x.split('億')[1].split('万')[1].strip('円'))) + '円' if '億' in x else x)
df2 = df2.apply(lambda x: x.split('万')[0] +'万0円' if '万円' in x else x)
df['B'] = df2.apply(lambda x: int(x.split('万')[0]) * 10000 + int(x.split('万')[1].split('円')[0]) if '万' in x else int(x.strip('円')))
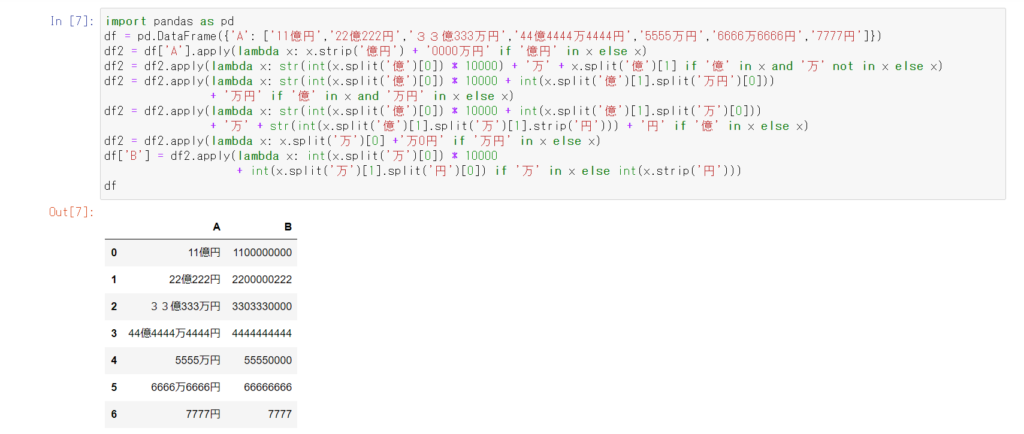
リンク
リンク